I’ve been doing tweetcarts (or sketches as I sometimes call them) for a while now. It’s really hard, for me at least, to come up with new ideas each week, but one thing I always like to go back on is trigonometry, specifically sine and cosine.
The key thing about sine and cosine is that the range is always [-1, 1]. We can use this to basically “modulate” anything that’s a number. For example, let’s make a blank PICO-8 project and have a circle drawn in the middle of the screen.
x=64
y=64
r=8
c=12
::_::
cls()
circfill(x,y,r,c)
flip()
goto _
Then, inside of the loop, we can modulate x by setting it to something like the following:
x = 64+16*cos(t())
and get this:
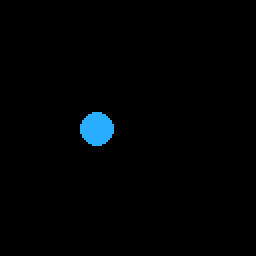
Note that t() is an alias for time(), which returns the amount of time since PICO-8 has started. What matters isn’t what t() returns, but the fact that cos(t()) will fluctuate between -1 and 1 since t() increases over time. As a result, x fluctuates between (64 – 16) and (64 + 16), or 48 to 80.
Now, if we do the same thing to y, but use sin() instead, we get a circular path! This happens because we’re actually dealing with polar coordinates, where x = radius * cos(angle) and y = radius * sin(angle).
y = 64+16*sin(t())
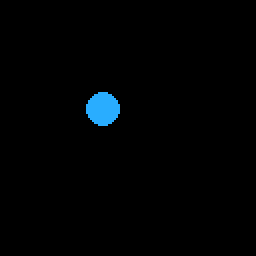
So what else can we modulate here? The circle has a radius, so we can modulate that too! By doing something like this:
r = 16+8*cos(t())
we get this:
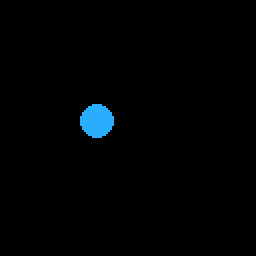
If you look closely, you’ll notice that the radius always seems to “align” with where the circle is at in its path. This happens because the angle being used for all 3 variables is the same. So, what if we made a separate variable for the radius? We’ll set it outside of the loop, then increment it inside of the loop.
x=64
y=64
r=8
c=12
a=0 --new angle variable for the radius
::_::
cls()
x = 64+16*cos(t())
y = 64+16*sin(t())
r = 16+8*cos(a)
circfill(x,y,r,c)
a+=0.05 --increment the new angle
flip()
goto _
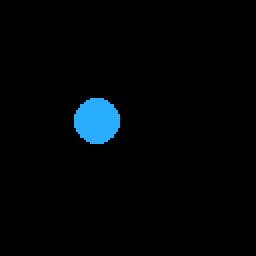
We can go even deeper with modulation. like modulating the x and y offsets, the color of the circle, or even the rate at which the angles are being incremented (although at that point it’s hard to tell how the end result changes).
After playing around with modulating numbers, I sometimes decide to draw multiple objects at different points in their modulation, or in other words, at different values in the angles being used.
x=64
y=64
r=8
c=12
a=0
::_::
cls()
for i=.1,1,.1 do
x = 64+32*cos(t()/5+i)
y = 64+32*sin(t()/5+i)
r = 12+6*cos(a)
circfill(x,y,r,c)
end
a+=0.05
flip()
goto _
Here, in addition to adjusting some of the numbers to make the end result look nicer, I added a for loop, from 0.1 to 1 in increments of 0.1 (since PICO-8’s trig functions use an input range of 0 to 1), and I add that to the angle being used for the x and y modulation. I’m also dividing t() by 5 to make x and y change at a slower rate. The end result is pretty cool.
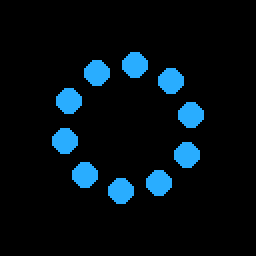
So, overall, modulation is really nice to experiment with. While it does sometimes make me focus too much on sinusodial-based things rather than try other methods of making art (bitwise art, fractals, randomness), it’s a pretty useful tool to have, and it can be used in various other scenarios as well.